Jupyter Notebooks for Engineering Classes
I have one more “traditional” engineering class in my time at Ohio State which is ECE 2020: Introduction to Analogue Circuits. I took digital circuits a couple semesters ago, and that class was basically boolean expressions but with little lines connecting to boxes. This class is more resistors/inductors/capacitors/I never took a class on complex numbers oh God. So, there’s a little more algebra involved, and it becomes especially unwieldy when we start using complex numbers for the phasor domain.
But I knew there were symbolic solvers out there (Wolfram Alpha, for one), and I was feeling more comfortable with Jupyter notebooks, so I decided to use Sympy to do all of my homework for ECE 2020 in a notebook.
Here’s how I set it up:
- Set up a virtual environment (preferably in Python3)
- Install iPython, Jupyter and Sympy
- Create a notebook
- Use Sympy and
cmath
to solve the hard problems
Setting up a Virtual Environment
Assuming you have python3
installed on your machine (if
you don’t, look here (or anywhere
on the internet) for instructions):
cd <your school folder>
python3 -m venv school-venv
. school-venv/bin/activate
Installing Dependencies
We need Jupyter and Sympy (Jupyter will install iPython as a dependency):
pip install jupyter sympy
Then we need to create a kernel for Jupyter that corresponds to this virtual environment:
. school-venv/bin/activate
ipython kernel install --user --name=school
Create a notebook
jupyter notebook <your school folder>
This will launch the web interface. From here, I navigate to my class folder and create a new notebook with my school kernel
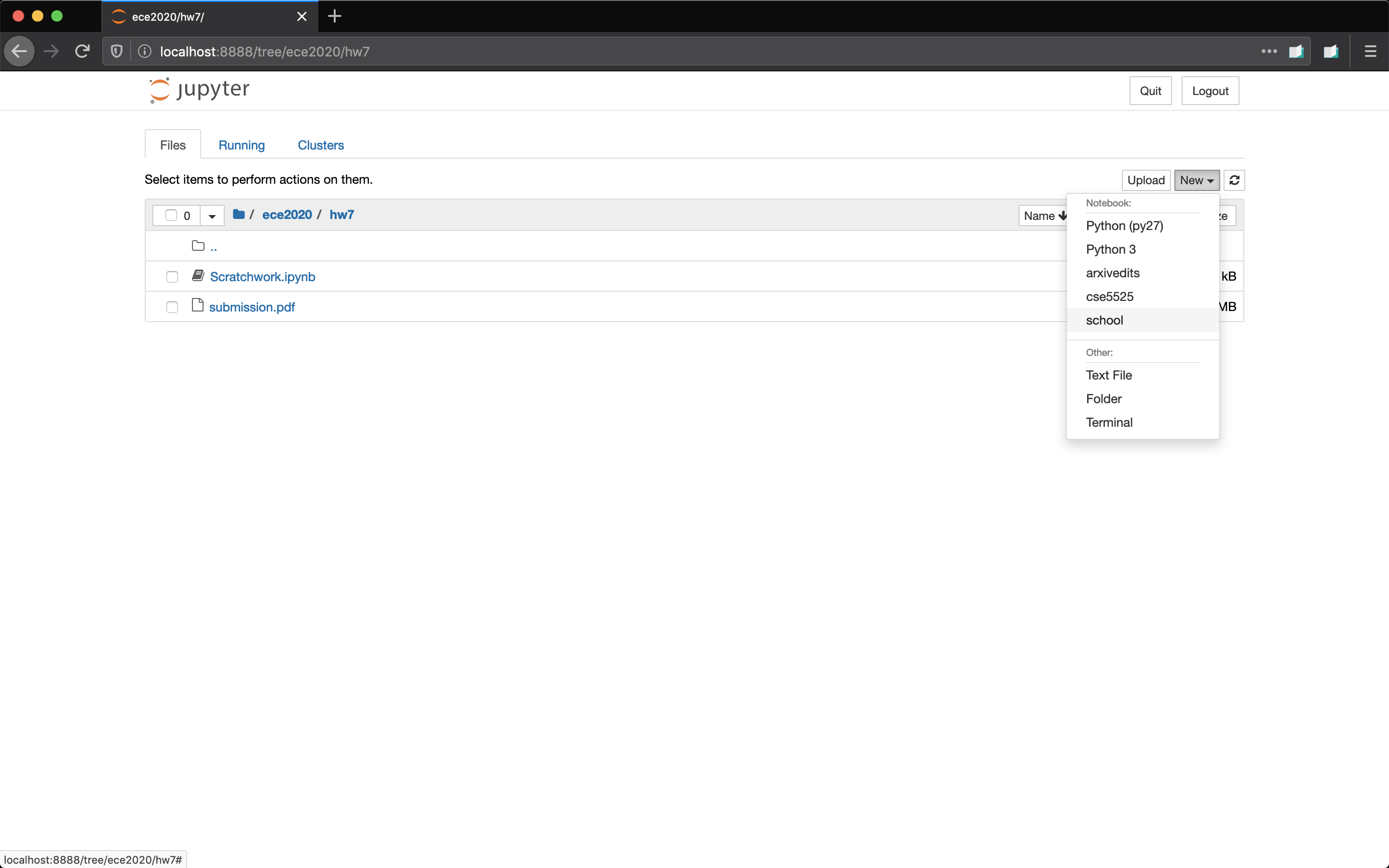
Solve Problems
I always import Sympy and exp
from cmath
,
and set up j to mean 0+1j
:
import sympy as sym
from cmath import exp
= 1j # for convenience j
And here’s an example of how I would do an ECE problem:
First, set up my constants.
= 300
w = 8
z1 = 8
z2 = 1+0j
v = 2+0j i
Next, I set up values that depend on constants.
= j * w * 3 * 10 ** -6
zL print('zL:', zL)
= 1 / (1/z1 + 1/(zL + z2))
zTH print('zTH:', zTH)
= -j / (300 * 5 * 10 ** -6)
zC print('zC:', zC)
Finally, I use Sympy to solve for variables in multiple equations.
Note: You need to set up
Va
andVc
assym.Symbol()
for Sympy to solve for it.
Note 2: The results from Sympy are not type
complex
; they must be cast before being used with othercomplex
numbers, or you end up with weird results.
= sym.Symbol('Va')
Va = sym.Symbol('Vc')
Vc
= sym.solve(
result
(- v) / z1 + (Va - Vc) / zL,
(Va - Va) / zL + Vc / z2 - i
(Vc # as far as I know, these equations are always assumed to equal 0
),
(Va, Vc)
)= complex(result[Va])
vTH print('vTH:', vTH)
And this is how it all might look within a Jupyter notebook:
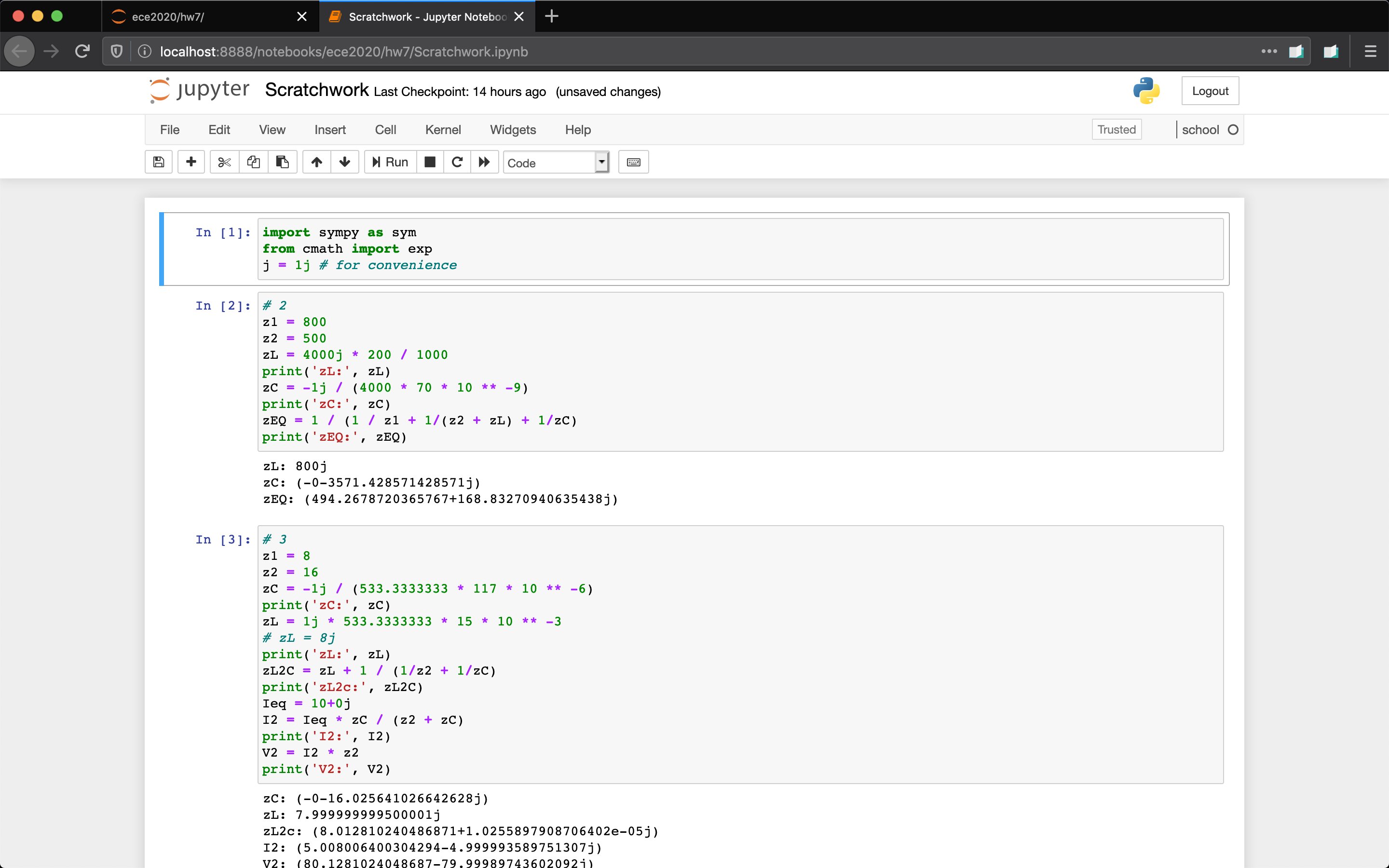
This is how I avoid doing any hard math in my ECE class.
Please email me if you have any comments or want to discuss further.
[Relevant link] [Source]
Sam Stevens, 2024